Define and apply an affine transform in 2D from control points
The same approach works for 3D points too.
Kaufmann, 2022.
[1]:
import numpy as np
import matplotlib.pyplot as plt
from shapely.geometry import Point, LineString
from shapely.affinity import affine_transform
from geometron.geometries import transforms as ggt
from geometron.plot import geometries as gpg
[2]:
origin_coords = [np.array([0.,0.]), np.array([0.,1.]), np.array([1.,0.]), np.array([1.,1.2])]
[3]:
destination_coords = [np.array([2.5,1.5]), np.array([2.,2.]), np.array([3.,2.]), np.array([2.5,3.])]
[4]:
transform_matrix, residuals, rank, singular = ggt.affine_transform_matrix(origin_coords, destination_coords)
[5]:
transform_matrix
[5]:
array([ 0.54508197, -0.45081967, 0.68032787, 0.69672131, 2.47540984,
1.40163934])
[6]:
origin_gcp = [Point(p) for p in origin_coords]
destination_gcp = [Point(p) for p in destination_coords]
transformed_gcp = [affine_transform(p, transform_matrix) for p in origin_gcp]
[7]:
ls = LineString([[1,1], [2,1], [1,4]])
Ls = affine_transform(ls, transform_matrix)
[8]:
fig, ax = plt.subplots()
for p in origin_gcp:
i = origin_gcp.index(p)
gpg.plot_shapely_obj(obj=p, ax=ax, color='g', marker='o', label='GCP - origin' if i == 0 else '_no_legend_')
for p in destination_gcp:
i = destination_gcp.index(p)
gpg.plot_shapely_obj(obj=p, ax=ax, markeredgecolor='r', markerfacecolor='r', marker='o', label='GCP - destination' if i == 0 else '_no_legend_')
for p in transformed_gcp:
i = transformed_gcp.index(p)
gpg.plot_shapely_obj(obj=p, ax=ax, markeredgecolor='k', markerfacecolor='w', marker='o', alpha=0.5, label='GCP - transformed' if i == 0 else '_no_legend_')
gpg.plot_shapely_obj(obj=ls, ax=ax, color='g', marker='x', label='object - origin')
gpg.plot_shapely_obj(obj=Ls, ax=ax, color='r', marker='x', label='object - transformed')
ax.axis('equal')
fig.legend(bbox_to_anchor=(1.25, 1), loc='upper right', borderaxespad=0)
[8]:
<matplotlib.legend.Legend at 0x7c87e7eefdf0>
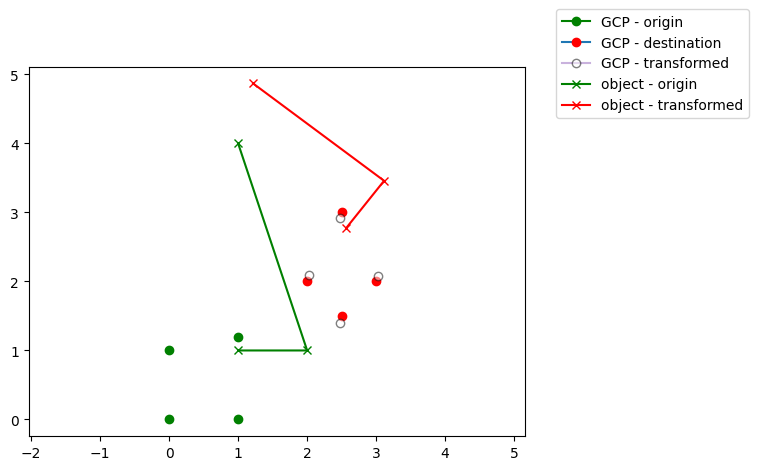